Been wanting to write an App for my phone since forever, before I even had a smartphone in fact, and nowadays had the note hanging on my whiteboard, judgingly looking at me.
This month (2019-09) I made this long had dream a reality.
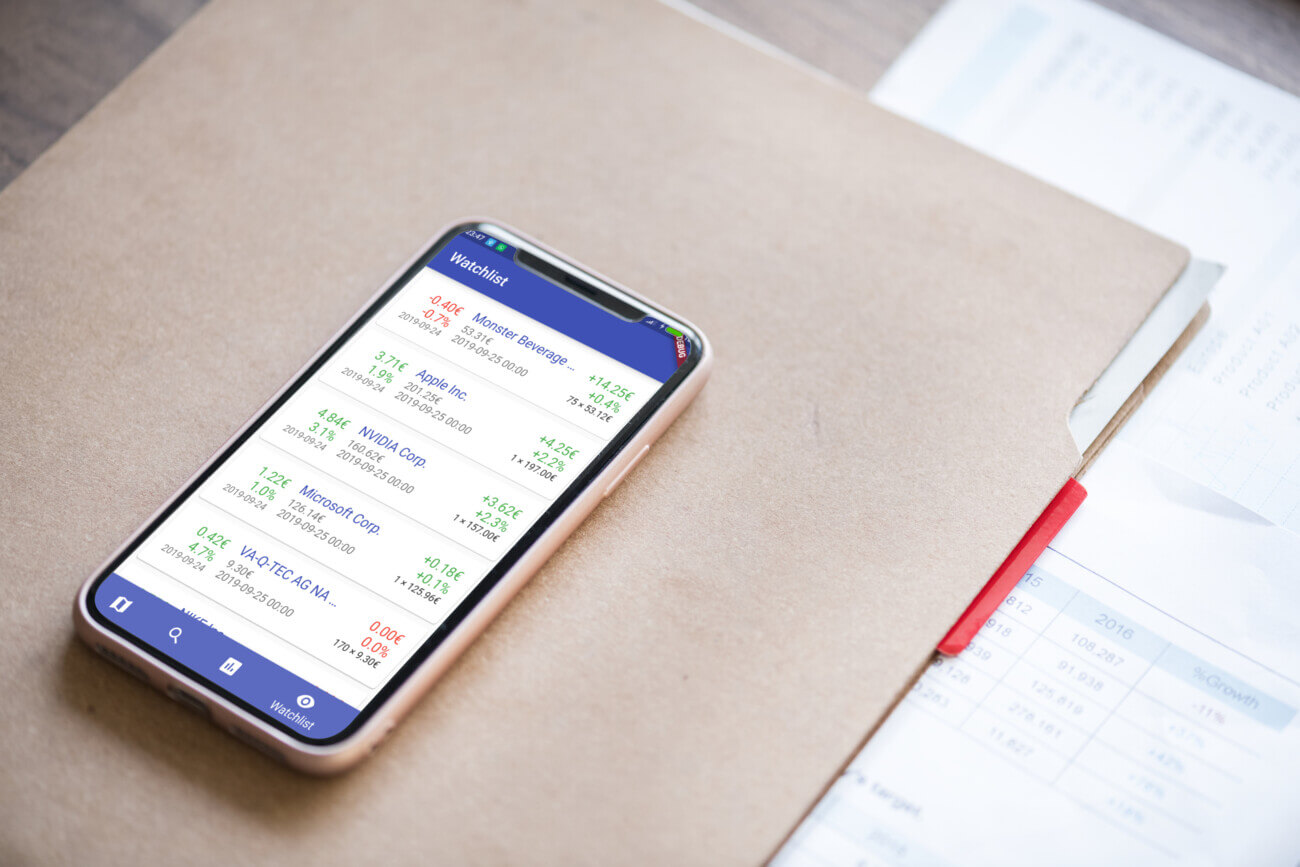
What pushed me over the edge to start this
Maybe some of you know that this year (2019) I started my stock trading journey, so I started using a couple different finance apps to easily catch up on news, my watchlist, quickly look up prices for different instruments, you get the idea.
In the beginning this was enough for me, I was only getting started so I didn't exactly know what I want to know, what I want to see, how I want to interact with all the information.
Soon I'll be trading for a whole year and know a lot more than in the beginning.
Now I want things, I expect things and I wish for things from my tools.
Like I said, I tried out a couple of different apps, narrowed them down and ended up with using the Finanzen.net-App as my daily driver. And before I continue I'd like to mention that the devs working on that app are probably really nice people and I hold no grudge against them personally.
Here it goes: I am greatly dissatisfied with the app's perfomance - so much so in fact, that this genuinely is the straw that broke the camels back.
Freezes, bugs, bad user experience, oh boy countless bugs, missing functionality, more bugs, weird behaviour and last but not least.. the bugs - did I mention those?
Like I said, I do not intend to shit on individual devs but that app currently is in a state that it makes me not want to use it anymore.
So I set out to prove to myself that I could do better.
Developing an app - where to start?
So the first step is to prove to myself that I can do it. I like to break such a problem down to a MVP or MVE, "Minimum Viable Product" or "Minimum Viable Experiment" respectively.
If you ever thought about making a product you know that feeling of having a million ideas for features and stuff you want to have, but this more often than not culminates in a half baked project that gets abandoned half way through because you don't make much progress and lose interest. (Been there, done that..)
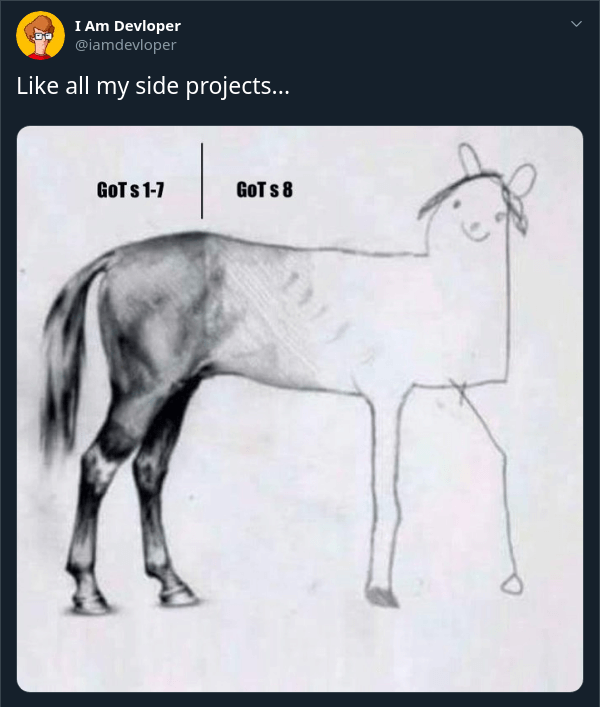
I found the key for me is to strive for a Minimally Viable Experiment.
Add all the stuff you think about to a backlog and strip out the most essential pieces, the core features that you need - not the ones you want. This is key. This helps you focus and to get something out the door. Don't spend time or at least don't spend much time on design. Make it work. A product that looks wonky, but works, still provides some value. A product that looks amazing but doesn't work is worthless.
Making the first prototype
I dove into Dart and Flutter instead of native Android Dev because I'd like to have the option to also release on iOS from the same codebase. Been seeing some talks at conferences about Flutter and it looked quite intriguing.
Read up on documentation, watch some tutorials, write code, read documentation, write some more code, watch some more tutorials, .. repeat over and over and then I got this:
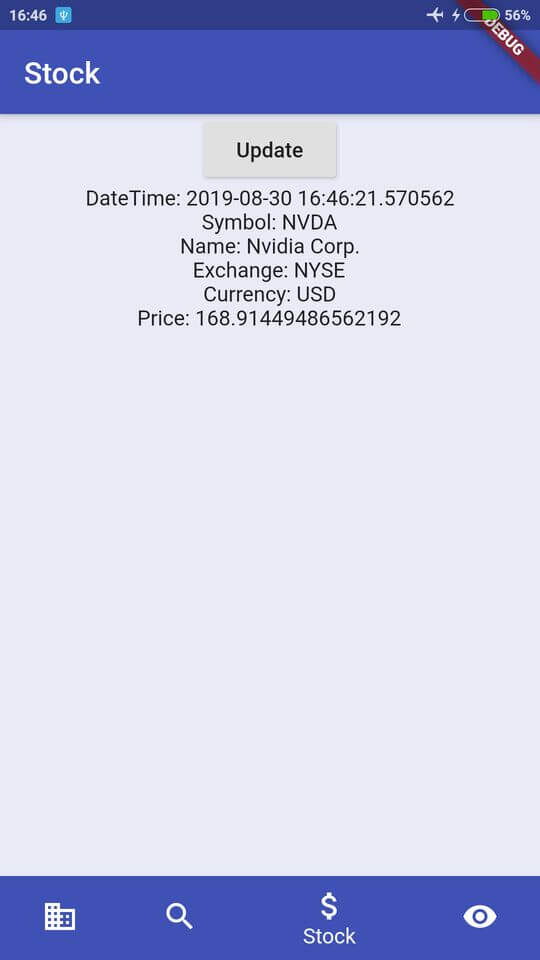
The funny thing here is.. nothing worked, haha. The displayed text is hardcoded because I'm still learning how to display anything on the screen. The button just displays the predefined text, that's it.
I decided that I probably would need three or four "Pages", so to speak.
- A dashboard for news, some sort of general analytics and the like
- A search page so that I can look up securities
- A place where I get detailed info about a security
- A watchlist page where I can save securities to and look them up later
Next, I needed a way to search for securities and I looked at several APIs and websites that provide that functionality. I narrowed multiple possibilities down to the REST API of Börse Frankfurt because it is a very well made site that responds with very easily parseable JSON.
As an example, if you search for 'test' they respond with objects that look like this:
{
"isin":"GB0031638363",
"wkn":null,
"symbol":null,
"investmentCompany":null,
"issuer":null,
"type":"EQUITY",
"typeName":{
"originalValue":"EQUITY",
"translations":{
"de":"Aktie",
"en":"Equity"
}
},
"count":0,
"name":{
"originalValue":"INTERTEK GROUP LS-,01",
"translations":{
"others":"Intertek Testing Services PLC"
}
}
}
Super duper comfortable to work with, hats off to you Börse Frankfurt.
Some tinkering later I got the very first search working:
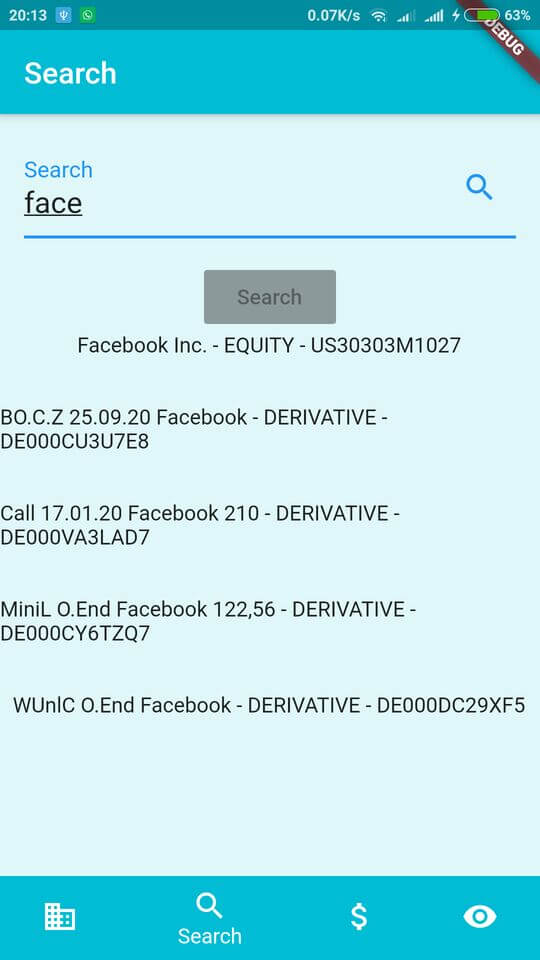
Next, I made the results into a list so that I can scroll down properly:
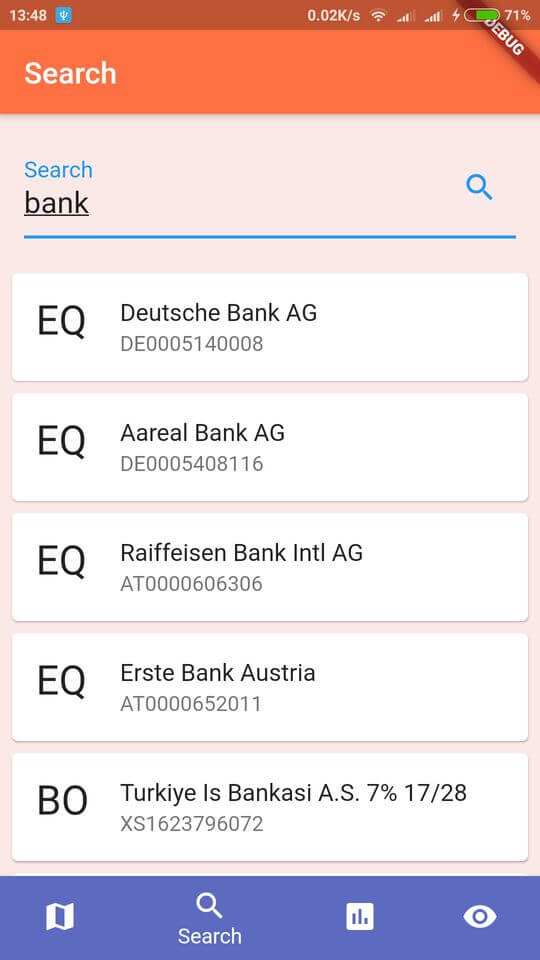
I created a local sqlite database that holds a couple tables. For example I store some search data, securities and watchlist items. Then I made it possible to save and display items in said watchlist:
![]() |
![]() |
After that there was some dynamically generated info when you look up a specific security:
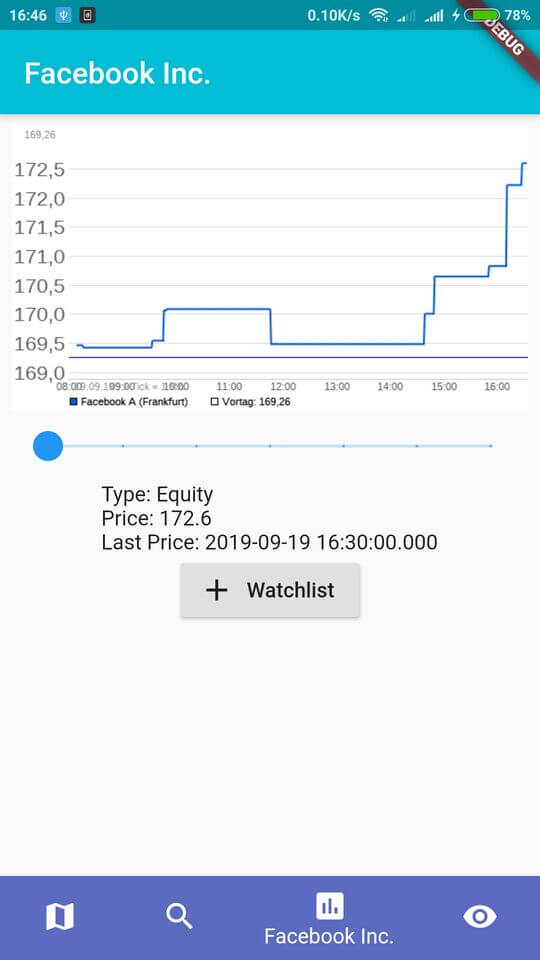
You see that Slider-Element under the chart? That let's you configure what chart you see from Intraday to one week, all up to ten years. That chart and slider is currently sort of just a placeholder before I implement a proper interactive chart.
In the name of prototyping quickly: Charts currently are just images that are being fetched from an API. Ultimately I'd like to implement charts where you can zoom in/out and show some very basic indicators. No actual trading will get done from the app so it doesn't have to be very comprehensive, some basic ones I'd like to have though.
Then I cleaned up the watchlist so that you get some useful data out of it:
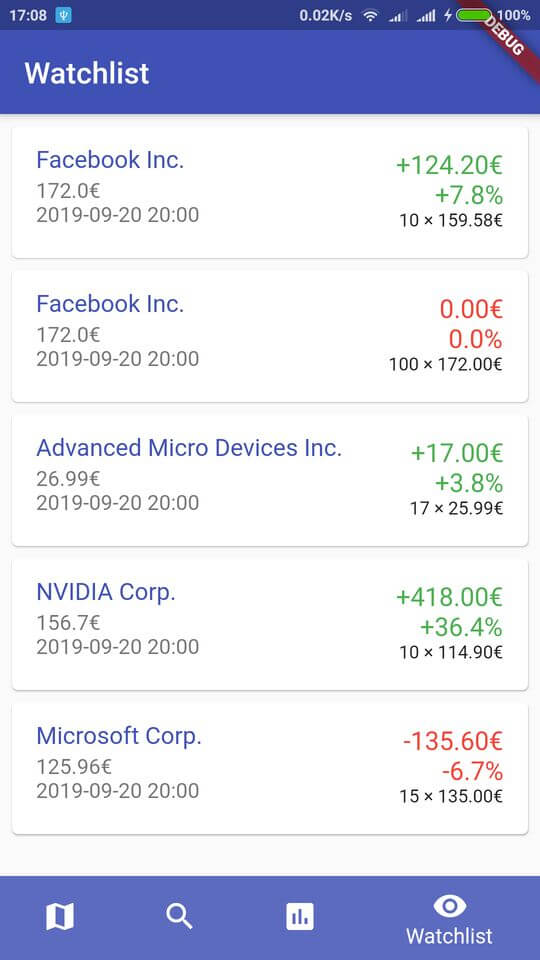
It starts to look like something actually usable, eh?
Add the difference to the last trading day so you can spot harsh changes. On the left you can see the previous trading day and the difference in absolute and percentage values. On the right you can see how much your position is currently worth.
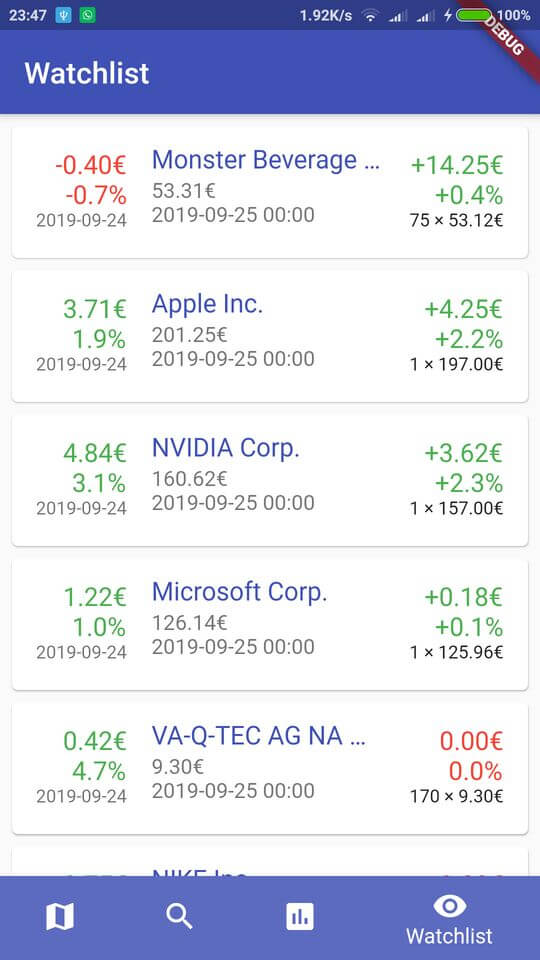
And with that shown, you now know the current state of my app.
- Search for securities
- Get some info about them
- Safe them to a watchlist
It works pretty well so far, so I think I have proven to myself that I indeed am capable of solving my needs and fulfilling my dream of making a (good) app. It is super simple but serves its purpose of being a rough, minimum viable experiment!
Problems
The more I laid out the structure and architecture of the app, I found it hard to get a really good overview of how the big picture is gonna look like and I found the way that data flows in Flutter pretty confusing. I heavily relied on Streams and the BLoC Pattern but that introduced many headaches you need to think about and left a bad taste in my mouth. Everything is so disjointed (which can be a good thing) but I found myself writing so much boilerplate code and spent way too much time thinking about the ways I need to work around the streams instead of working on logic for my app that I got frustrated in the end.
Don't get me wrong though, Streams are a cool concept and I see the value in them. Now in hindsight I'd build the app in a different way though. I think it'd be considerably easier with a single source of truth like a Redux Store.
This is why next time you're in for a treat! We're gonna get technical.
What's next
Please keep in mind that this article-series is just for fun and won't be receiving consistent updates. It'll probably be quite a while until the next installment.
I generally plan to release posts after I fixed some bugs, improved the code and implemented something new that's worth showing. This ensures that every post is interesting on it's own instead of little updates that noone really cares about. For this post I let pretty much all of the technical details out and didn't show code. I think I want to change that up next time. Ready yourselves to dive into some code ;)
Did you enjoy this?
Please reach out and tell me what you think. Would you like to see more, would you like to see something implemented or are you interested in something specific?
Thanks for your time, cheers.
- Daniel Biegler
Was denkst du?